In this post, I’ll guide you through setting up a solution to manage Oracle Cloud Infrastructure (OCI) instances directly from Home Assistant using HTTP calls. By combining the power of the oci
CLI and a Node.js server, you’ll be able to start, stop, and monitor your OCI instances effortlessly.
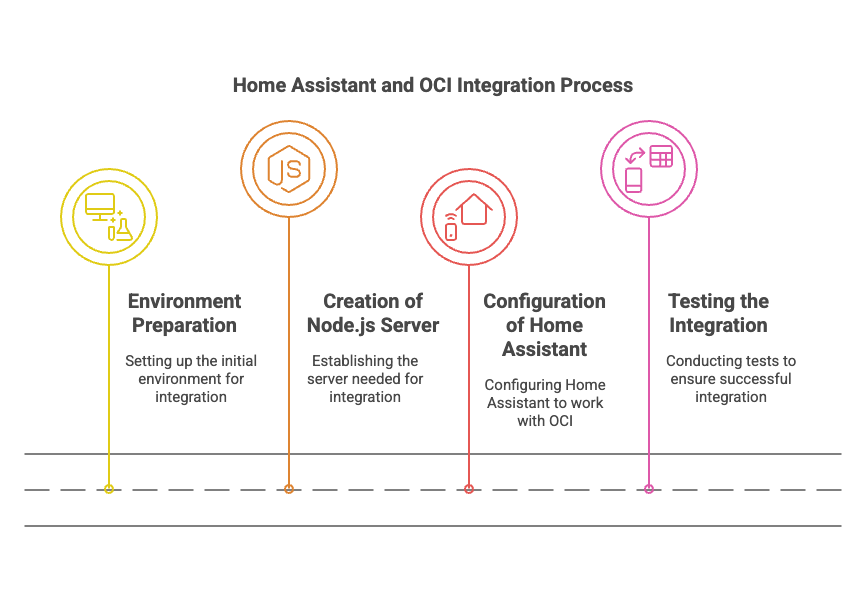
Requirements
Before starting, ensure you have the following:
- Home Assistant installed and running.
- A server with Node.js and OCI CLI installed.
- OCI CLI configured with the appropriate credentials.
- Your instance’s OCID for testing purposes.
- Basic knowledge of editing YAML files in Home Assistant.
Logical Architecture
Let’s visualize how the components interact:
- Home Assistant sends HTTP requests using its
rest_command
feature. - Node.js server receives these requests, executes OCI CLI commands, and responds with results.
- OCI performs the requested actions (start, stop, or retrieve instance status).
- Home Assistant displays the instance status using a sensor.
Setting Up the Node.js Server
- Install Node.js on your server.
sudo apt update
sudo apt install nodejs npm -y
npm install express body-parser
- Install OCI CLI by following OCI CLI documentation.
- Create the Node.js script (sudo nano oci_instances.js):
const express = require('express');
const bodyParser = require('body-parser');
const { exec } = require('child_process');
const app = express();
const port = 3000;
app.use(bodyParser.json());
app.post('/instance-action', (req, res) => {
const { action, instanceId } = req.body;
if (!action || !instanceId) {
return res.status(400).json({ error: 'Action and instanceId are required' });
}
const command = `oci compute instance action --action ${action} --instance-id ${instanceId}`;
console.log(`Executing command: ${command}`);
exec(command, (error, stdout, stderr) => {
if (error) {
console.error(`Error: ${error.message}`);
return res.status(500).json({ error: error.message });
}
if (stderr) {
console.error(`Stderr: ${stderr}`);
return res.status(500).json({ error: stderr });
}
res.json({ message: 'Command executed successfully', output: stdout });
});
});
app.post('/instance-status', (req, res) => {
const { instanceId } = req.body;
if (!instanceId) {
return res.status(400).json({ error: 'Instance ID is required' });
}
const command = `oci compute instance get --instance-id ${instanceId}`;
exec(command, (error, stdout, stderr) => {
if (error) {
return res.status(500).json({ error: error.message });
}
if (stderr) {
return res.status(500).json({ error: stderr });
}
try {
const data = JSON.parse(stdout);
const status = data.data['lifecycle-state'] || 'UNKNOWN';
res.json({ instanceId, status });
} catch (parseError) {
res.status(500).json({ error: 'Failed to parse OCI response', details: parseError.message });
}
});
});
app.listen(port, () => {
console.log(`Server listening on port ${port}`);
});
- Run the server:
node oci_instances.js
Configuring Home Assistant
- Edit
configuration.yaml
: Add the followingrest_command
and sensor configurations.
rest_command:
start_instance:
url: "http://nodejs_server__ipaddress:3000/instance-action"
method: POST
headers:
Content-Type: "application/json"
payload: >
{
"action": "START",
"instanceId": "ocid1.instance.oc1.example-region.exampleuniqueID"
}
stop_instance:
url: "http://nodejs_server__ipaddress:3000/instance-action"
method: POST
headers:
Content-Type: "application/json"
payload: >
{
"action": "STOP",
"instanceId": "ocid1.instance.oc1.example-region.exampleuniqueID"
}
sensor:
- platform: rest
name: OCI Instance Status
resource: "http://nodejs_server__ipaddress:3000/instance-status"
method: POST
headers:
Content-Type: "application/json"
payload: >
{
"instanceId": "ocid1.instance.oc1.example-region.exampleuniqueID"
}
value_template: "{{ value_json.status }}"
scan_interval: 60
unique_id: "oci_instance_status_example"
- Restart Home Assistant: Save your changes and restart
- Create automations: You can now automate instance management by triggering these commands from Home Assistant’s automation editor.
Testing the Integration
- Open Home Assistant and call the
rest_command.start_instance
service. - Check if the instance starts in the OCI console.
- Verify the status with the sensor after a few seconds.
Conclusion
This integration bridges smart home technology and cloud infrastructure management. With Node.js as the middleware, Home Assistant can execute complex cloud operations, offering endless automation possibilities for OCI users. Experiment with adding more commands or combining this with other tools to expand functionality further!